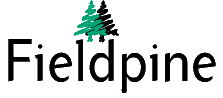
Example: Minimal User Interface
This page is using older replaced techniques and needs to be revised. The overall concept is still the same but some of the code itself is now different
This example shows a minimal User Interface that might be used. This example hardcodes a few options in the sake of simplicity. This is an intentionally simple example that does the following
- Displays a web page
- Has two quicksell buttons for "Milk" or "Bread"
- Has the option to void the sale, or complete with cash
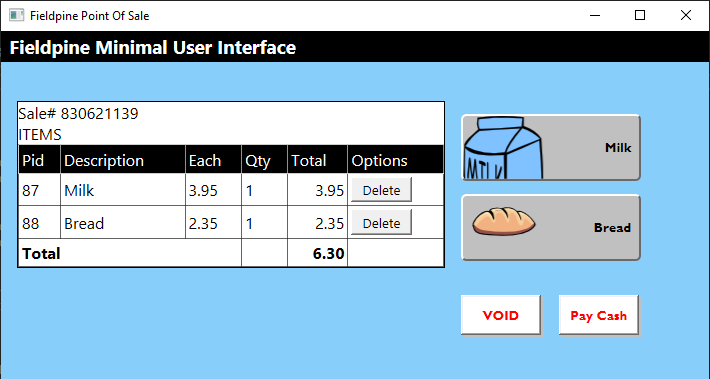
This user interface was created with the following HTML. The vast majority of this file is CSS to provide the styling, which we have included inline for this example, but would normally be in a seperate file.
The Key parts that relate to interfacing with Fieldpine are highlighted
<!DOCTYPE html> <html lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <title>Minimal Example</title> <style> * { font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif; font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif; } .milk { background: url(https://fieldpine.com/geni/clipart_2312.png) no-repeat; background-color: inherit; } .bread { background: url(https://fieldpine.com/geni/clipart_2332.png) no-repeat; background-color: inherit; } #salelist { background-color: white; resize: both; overflow: auto; margin-left: 1em; } #salelist thead { background-color: black; color: white; } #salelist tfoot { font-weight: 700; } .abutton { width: 13.5em; height: 5em; overflow: hidden; max-width: 15em; background-color: silver; border-radius: 0.4em; margin-right: 1em; margin-top: 1em; text-align: right; font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif; font-weight: 700; } .paybutton { width: 6em; height: 3em; overflow: hidden; max-width: 10em; background-color: white; color: red; box-shadow: silver 0.2em 0.2em; margin-right: 1em; margin-top: 1em; text-align: center; font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif; font-weight: 700; } #salelist { min-height: 8em; border: 1px solid black; } #mainopts { float: right; display: inline-block; max-width: 35%; margin-left: 1em; } </style><script src="https://fieldpine.com/report/elink/webui.js"></script> // Functions to interact with Fieldpine POS <script> function SaleListEvent() { // This function receives the SaleObject whenever it changes and draws it to the web page, using whatever styling you require var sLastSale = Fieldpine.LastSale; var a = new Array; if ((typeof sLastSale.data !== 'undefined') && (sLastSale.data !== null) && (typeof sLastSale.data.ReceiptNo !== 'undefined')) { a.push("Sale# "); a.push(eLink_ExtractStr(sLastSale, "data.ReceiptNo", "?")); if (typeof sLastSale.data.LINE !== 'undefined') { a.push("<BR>ITEMS<table border=1 cellpadding=3 style='border-collapse:collapse' width='100%'>"); a.push("<thead><tr><td>Pid</td><td>Description</td><td>Each</td><td>Qty</td><td>Total</td><td>Options</td></tr></thead>"); a.push("<TBODY>"); for (var k = 0; k < sLastSale.data.LINE.length; k++) { var rec = sLastSale.data.LINE[k]; a.push("<tr><Td>"); a.push(eLink_ExtractStr(rec, "Pid", "")); a.push("</td><Td>"); a.push(eLink_ExtractStr(rec, "Description", "")); a.push("</td><Td>"); a.push(eLink_ExtractStr(rec, "UnitPrice", "")); a.push("</td><Td>"); a.push(eLink_ExtractStr(rec, "Qty", "")); a.push("</td><Td align='right'>"); a.push(eLink_NiceFormat(eLink_ExtractNum(rec, "TotalPrice", 0), 2)); a.push("</td><Td>"); a.push("<input type='button' value='Delete' onclick='FieldpinePrimitive(\":sale delete line sequence "); a.push(eLink_ExtractStr(rec, "Seqnce", "")); a.push("\")'>"); a.push("</td></tr>"); } a.push("</TBODY>"); a.push("<tfoot>"); a.push("<tr><td colspan=3>Total</td><td>"); a.push("</td><td align='right'>"); a.push(eLink_NiceFormat(eLink_ExtractNum(sLastSale.data, "Total", 0), 2)); a.push("</td><td>"); a.push("</td></tr>"); a.push("</tfoot>"); a.push("</table>"); } } if (a.length === 0) a.push("Waiting for Sale"); document.getElementById("salelist").innerHTML = a.join(""); } </script></head> <body style="margin:0;background-color:lightskyblue"> <div style="background-color:black;width:100%;padding:0.2em;margin-bottom:2.4em;color:white"> <h3 style="padding:0;margin:0"> Fieldpine Minimal User Interface</h3> </div> <div id="mainopts"> <input type="button" class="abutton milk" value="Milk" onclick="FieldpinePrimitive(':sale add line pid 87')" /> <input type="button" class="abutton bread" value="Bread" onclick="FieldpinePrimitive(':sale add line pid 88')" /> <br /><br /> <input type="button" class="paybutton" value="VOID" onclick="FieldpinePrimitive(':sale phase void')" /> <input type="button" class="paybutton" value="Pay Cash" onclick="FieldpinePrimitive(':sale pay type cash amount all')" /> </div> <div id="salelist"> <Center><br /><br />Salelist</Center> </div> <script> Fieldpine.EventHandle.addEventListener('FieldpineSaleChange', SaleListEvent); </script> </body> </html>