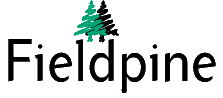
WebView2 Barcode Handling
Barcode scanners come in a wide range of different interfaces and not all of these can be easily controlled by the webpage. A further complication is that barcode scanners may send the same barcode in varying formats depending on how the scanner itself is configured. For this reason, WebView screens do not try and directly trap or contain the barcode scanner, instead relying on messages from PosGreen.
Bypassing PosGreen
Often, you may need to capture a barcode without letting the POS attempt to decode it first. If you are currently displaying a prompt in HTML asking for a serial number you might want the user to be able to scan that instead of typing.
PosGreen sends all barcodes received to the WebView2 page first via a PostMessage. You can trap these messages and accept or decline them. If you do not send any form of response, or take too long to reply, the Pos assumes you did not process it and continues.
If you have an input field as shown
<input type="text" id="serialnumberinputfield" placeholder="Enter serial number here - tip, you can use a barcode scanner" />
Then the following Javascript informs PosGreen you are accepting raw barcodes. The "serialnumberinputfield" is your reference string and sent back to you when barcodes are scanned
window.chrome.webview.postMessage("ui:barcode,acceptraw,serialnumberinputfield");
When you are finished accepting barcodes, the following removes your registration
window.chrome.webview.postMessage("ui:barcode,removeraw,serialnumberinputfield");
When you are actively registered, the POS sends a message to you for each barcode scan. You need code similar to the following to register to receive these messages and also to process them
// Register we want messages. You almost certainly already have this somewhere already window.chrome.webview.addEventListener('message', MessageFromFieldpinePos); // When we receive a message, see if barcode scan and route to our input field function MessageFromFieldpinePos(event) { if (typeof event.data === 'object') { if (event.data.Type === 'barcodescan') { // Our reference string is actually the fields DOM ID let trg = document.getElementById(event.data.CallerParam); if (trg) { // Post back to PosGreen saying we took/used the barcode and it should not process any further window.chrome.webview.postMessage("advise:accept," + event.data.Id); trg.value = event.data.data.RawScan; } else { // Post back to PosGreen saying we didn't use this barcode, so continue with normal processing window.chrome.webview.postMessage("advise:reject," + event.data.Id); } } } }
Complete Small Example
<head> <script> // Register we want messages. You almost certainly already have this somewhere already window.chrome.webview.addEventListener('message', MessageFromFieldpinePos); // When we receive a message, see if barcode scan and route to our input field function MessageFromFieldpinePos(event) { if (typeof event.data === 'object') { if (event.data.Type === 'barcodescan') { // Our reference string is actually the fields DOM ID let trg = document.getElementById(event.data.CallerParam); if (trg) { // Post back to PosGreen saying we took/used the barcode and it should not process any further window.chrome.webview.postMessage("advise:accept," + event.data.Id); trg.value = event.data.data.RawScan; } else { // Post back to PosGreen saying we didn't use this barcode, so continue with normal processing window.chrome.webview.postMessage("advise:reject," + event.data.Id); } } } } </script> </head> <body> <p>Scan a barcode <input size=20 id='testbarcode'> <script> // Tell Pos we are actively processing barcodes now window.chrome.webview.postMessage("ui:barcode,acceptraw,testbarcode"); </script> </body>